ImGui
This is yet another continuation of my game engine project (Part 1, Part 2)
This week I wanted to check out ImGui which is a nice C++ GUI library targeted towards use in internal tools and embedded systems.
I've known about ImGui for a couple years now but never used it before.
Before I could start integrating ImGui I needed to finish my work from last week.
I had started implementing my postprocessing stack but never actually finished it.
I last left it in a weird state where I could only use one postprocessing effect at a time, using zero or more would crash the application
(The reason for this is that I hadn't implemented proper double buffering, and made an assumption about which buffer the result was rendered in).
With this out of the way I get started with ImGui, and I was pleasantly surprised at how easy it was!
I spent more time fighting with visual studio than fighting with the actual library (vs refused to recompile objs.. whyyyyy,,,,)
Once I had a basic example running I just integrated it into my game loop and almost got it going to the first try.
After this I spent most of my time just studying the provided samples and copy-pasting writing simple editor windows. It's quite satisfying to see it all coming together!
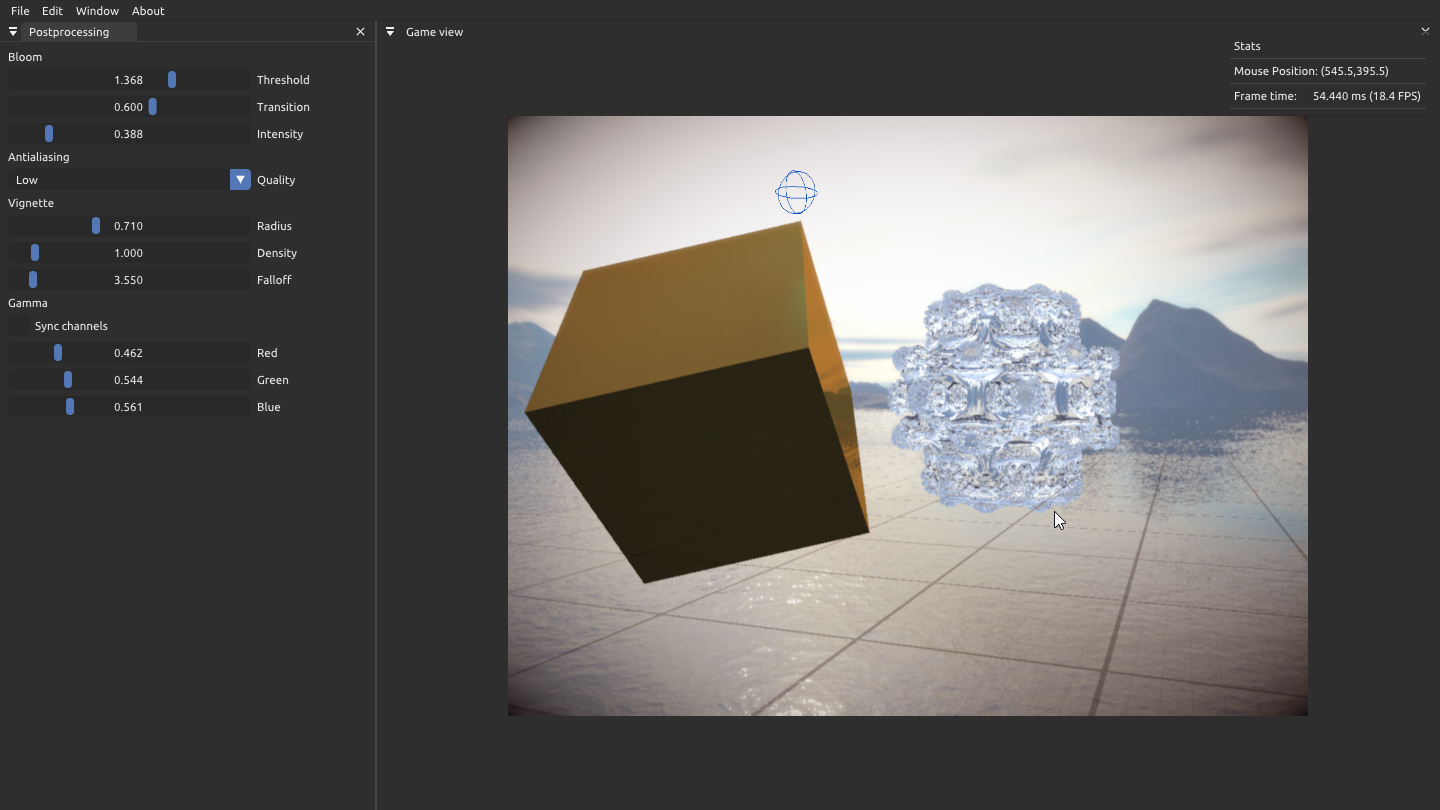
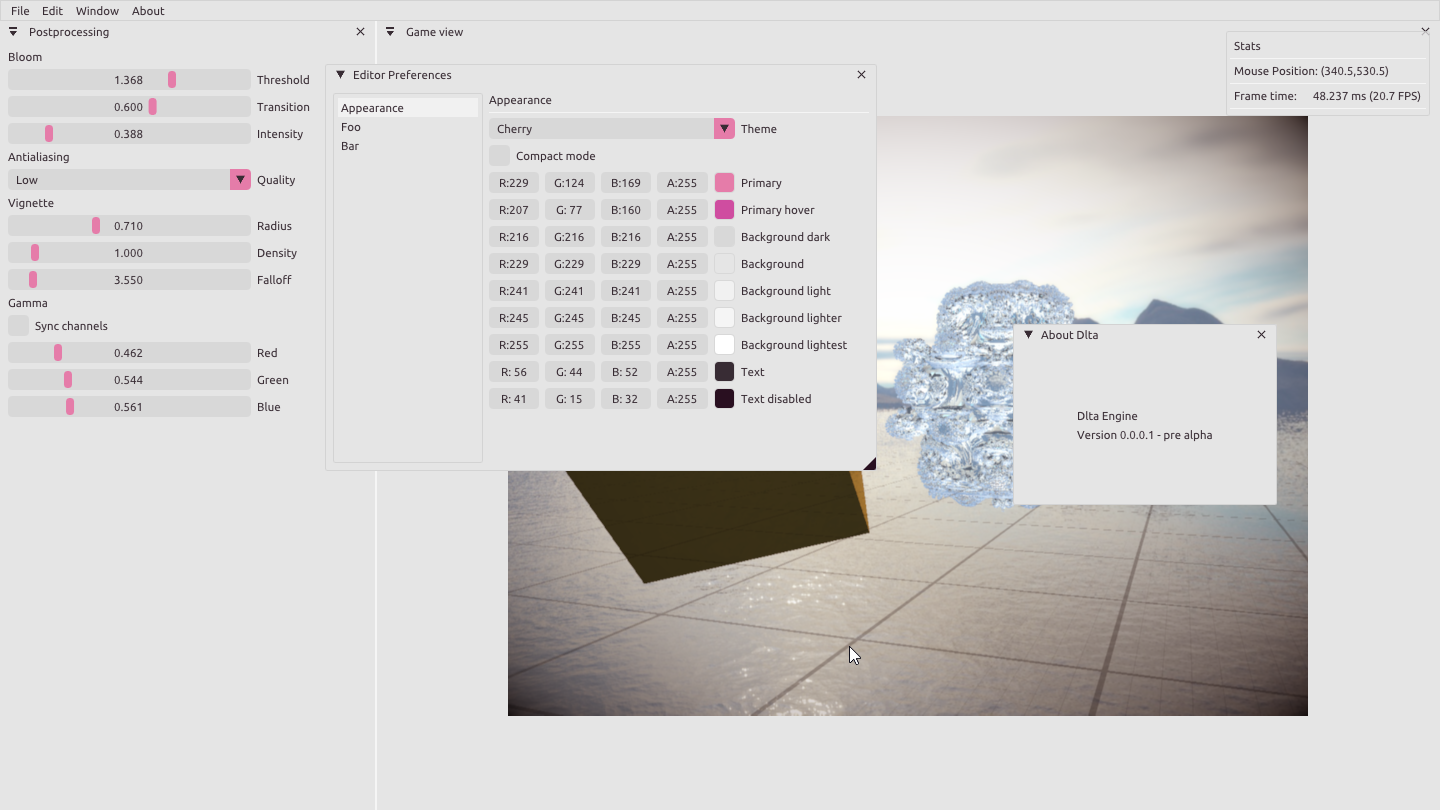
Not only does it look good, actually creating the gui is also incredibly easy.
I would like to share a complete example of how my vignette is currently implemented, GUI code and all.
C++:
class Vignette : public Effect {
public:
float radius = 1.;
float density = 1.;
float falloff = 2.;
Vignette() : Effect("vignette", "Vignette") { }
void onEditorGui() {
ImGui::SliderFloat("Radius", &radius, 0, 2);
ImGui::SliderFloat("Density", &density, .1f, 10);
ImGui::SliderFloat("Falloff", &falloff, 1., 32);
}
void onBind() {
shader.setFloat("radius", radius);
shader.setFloat("density", density);
shader.setFloat("falloff", falloff);
}
};
Shader:
out vec4 FragColor;
in vec2 TexCoords;
uniform sampler2D screenTexture;
uniform float radius;
uniform float density;
uniform float falloff;
void main()
{
vec2 center = TexCoords-.5;
vec2 uv = TexCoords;
// ugly aspect ratio fix
uv.x*=800./600.;
vec3 col = texture(screenTexture, TexCoords).rgb;
float vignette = 1-max(0,pow(length(center)/radius, falloff) * density);
col *= vignette;
FragColor = vec4(col,1);
}